일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- VR
- 개발
- 유니티 GUI
- Oculus
- 포트폴리오
- Photon Fusion
- 팀프로젝트
- 가상현실
- XR
- 유니티
- HAPTIC
- 앱 배포
- 오브젝트 풀링
- input system
- meta
- 개발일지
- 멀티플레이
- 길건너 친구들
- OVR
- 드래곤 플라이트
- 드래곤 플라이트 모작
- 유니티 UI
- 유니티 Json 데이터 연동
- 팀 프로젝트
- meta xr
- CGV
- ChatGPT
- 모작
- 오큘러스
- 연습
Archives
- Today
- Total
EasyCastleUNITY
Hero Shooter 새로운 Input System 활용한 조이스틱 이동 본문
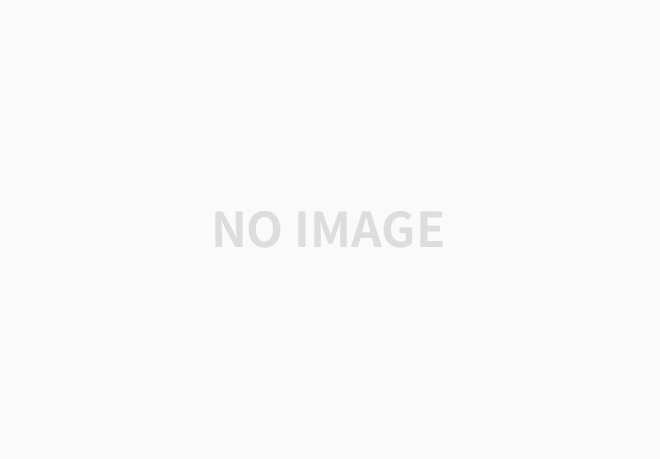
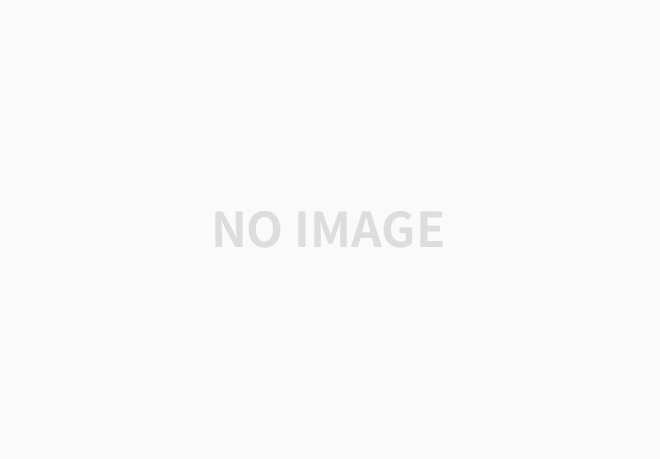
On-Screen Stick 컴포넌트에서 경로를 지정해야 한다.
Player Input 컴포넌트의 Behavior는 Invoke C Sharp Event를 사용한다.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.InputSystem;
public class TestInputManager : MonoBehaviour
{
[SerializeField] Transform playerTransform;
private Vector3 moveDir;
private Animator anim;
private PlayerInput playerInput;
private InputActionMap mainActionMap;
private InputAction moveAction;
private void Start()
{
this.anim = this.playerTransform.gameObject.GetComponent<Animator>();
this.playerInput = this.GetComponent<PlayerInput>();
this.mainActionMap = this.playerInput.actions.FindActionMap("PlayerActions");
this.moveAction = this.mainActionMap.FindAction("Move");
this.moveAction.performed += (context) => {
Debug.Log("performed");
Vector2 dir = context.ReadValue<Vector2>();
this.moveDir = new Vector3(dir.x, 0, dir.y);
this.anim.SetInteger("State", 1);
};
this.moveAction.canceled += (context) =>
{
Debug.Log("canceled");
this.moveDir = Vector3.zero;
this.anim.SetInteger("State", 0);
};
}
private void Update()
{
if(this.moveDir != Vector3.zero)
{
this.playerTransform.rotation = Quaternion.LookRotation(this.moveDir);
this.playerTransform.Translate(Vector3.forward * Time.deltaTime * 4.0f);
}
}
#region SEND_MESSAGE
public void OnMove(InputValue value)
{
Vector2 dir = value.Get<Vector2>();
this.moveDir = new Vector3(dir.x, 0, dir.y);
Debug.Log("SEND_MESSAGE 실행");
Debug.Log(dir);
this.anim.SetInteger("State", 1);
}
#endregion
#region UNITY_EVENTS
public void OnMove(InputAction.CallbackContext context)
{
Vector2 dir = context.ReadValue<Vector2>();
this.moveDir = new Vector3(dir.x, 0, dir.y);
Debug.Log("UNITY_EVENTS 실행");
Debug.Log(dir);
this.anim.SetInteger("State", 1);
}
#endregion
}
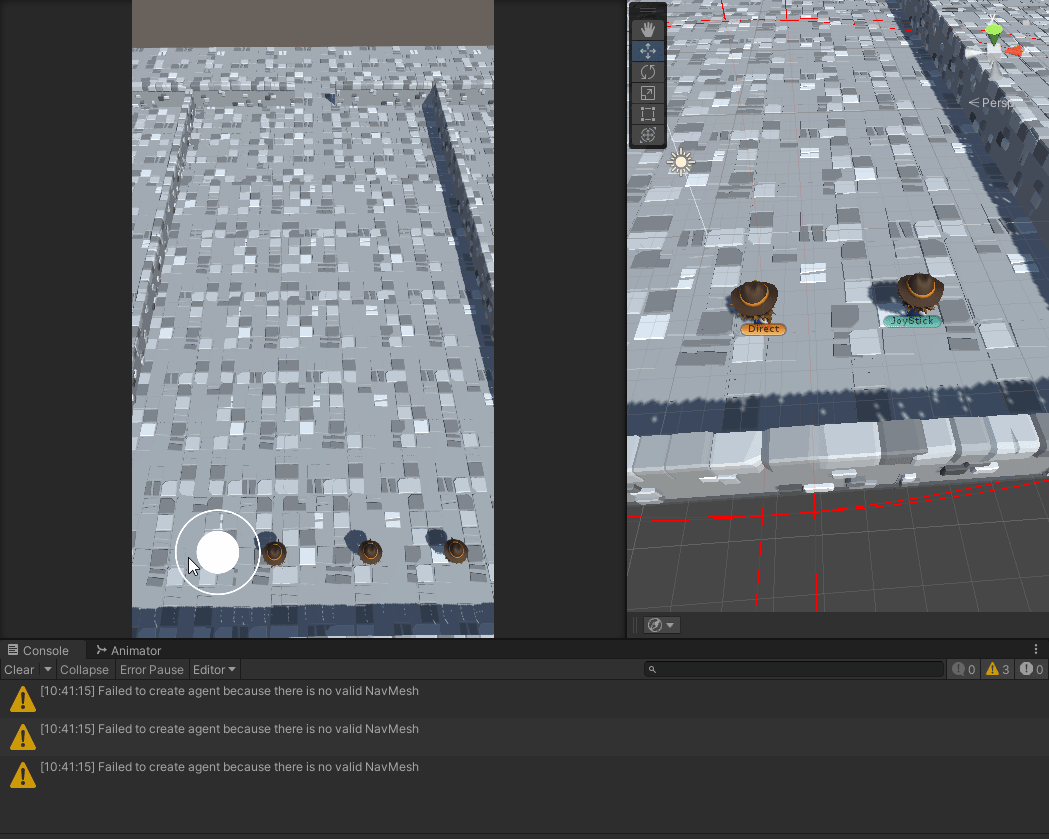
'유니티 심화' 카테고리의 다른 글
Hero SHooter 개발일지 2 (0) | 2023.09.03 |
---|---|
유니티 데이터 연동 (0) | 2023.09.01 |
Hero Shooter new Input System Test (0) | 2023.08.31 |
HeroShooter 개발일지1 (0) | 2023.08.30 |
HeroShooter 중간과정 정리 (0) | 2023.08.29 |